Following a recent SharePoint 2007 to 2010 upgrade, we got a report of an RSS Viewer that was not displaying any content.
The message shown was “The requested RSS feed could not be displayed. Please verify the settings and url for this feed. If this problem persists, please contact your administrator.”

After confirming for myself, I checked the feed that the RSS Viewer was pulling, and found it was from the Cisco support forums at https://supportforums.cisco.com/community/feeds/video?community=2004.
Next we checked the SharePoint Logs to find the following entry:
04/02/2012 10:17:05.24 w3wp.exe (0x4610) 0x3D3C SharePoint Portal Server Web Parts 8imh High RssWebPart: Exception handed to HandleRuntimeException.HandleException System.Net.WebException: The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel. —> System.Security.Authentication.AuthenticationException: The remote certificate is invalid according to the validation procedure. at System.Net.Security.SslState.StartSendAuthResetSignal(ProtocolToken message, AsyncProtocolRequest asyncRequest, Exception exception) at System.Net.Security.SslState.ProcessReceivedBlob(Byte[] buffer, Int32 count, AsyncProtocolRequest asyncRequest) at System.Net.Security.SslState.StartReceiveBlob(Byte[] buffer, AsyncProtocolRequest asyncRequest) at System.Net.Security.SslState.ProcessReceivedBlob(Byte[] buffer, Int32 count, AsyncProtocolRe… 193b14f8-f282-438d-bf91-36bb7c640d03
A corresponding entry in the Event log was also a clue.
An operation failed because the following certificate has validation errors:\n\nSubject Name: CN=supportforums.cisco.com, OU=Communications and Collaboration Team, O=Cisco Systems, L=San Jose, S=CALIFORNIA, C=US\nIssuer Name: CN=VeriSign Class 3 Secure Server CA – G3, OU=Terms of use at https://www.verisign.com/rpa (c)10, OU=VeriSign Trust Network, O=”VeriSign, Inc.”, C=US\nThumbprint: CAF5027FDDE630D4AC3FFB1000FC2E09B18249A3\n\nErrors:\n\n The root of the certificate chain is not a trusted root authority..
What was going on?
The Cisco site had a chain of SSL certificates that was 3 layers deep.
- A root certificate
- An intermediate or secondary certificate
- A site certificate

As the RSS Viewer attempted to connect to this location, it connects as the SharePoint service, not as a browser. SharePoint then tried to travel up the certificate chain to confirm the authenticity of each layer. When it got to the intermediate certificate, it choked, not able to validate it as a legitimately trusted source.
How do we fix it?
In order for SharePoint to recognize the Verisign certificates chained together and used by the Cisco site, we need to explicitly tell SharePoint they are trustworthy. Thankfully, there is a simple to use UI as part of Central Administration where we can do just that.
First, log in to Central Administration, then click on the ‘Security’ menu item.
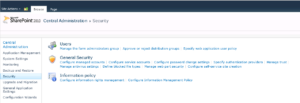
Then, select ‘Manage Trust’ from the ‘General Security’ section.
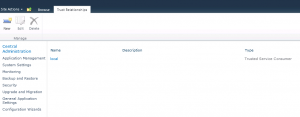
Before we can add the Verisign certificates as trusted relationships, we have to retrieve them from the Verisign website. All of Verisigns root and secondary certificates can be found at http://www.verisign.com/support/roots.html
By examining the certificate details in the Browser, we can determine which root certificate to download and add to SharePoint. In our case, there needed root was:
Root 3
VeriSign Class 3 Primary CA – G5
Description: This root CA is the root used for VeriSign Extended validation Certificates and should be included in root stores. During Q4 2010 this root will also be the primary root used for all VeriSign SSL and Code Signing certificates.
Download the Root Certificate file.
Now, add the Trust Relationship to SharePoint
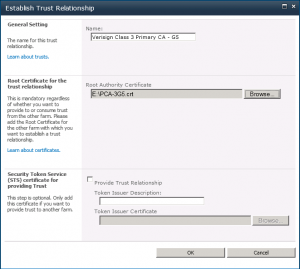
After that certificate is in place, you can return to the page with the RSS Viewer and confirm it is able to retrieve the results.

If not, then there may be additional certificates in the chain that you would need to retrieve and add to SharePoint.
The Trust Relationships in SharePoint are also used when calling out to an external web service from a web part.
Thanks to http://blog.mattsampson.net/index.php/calling-a-webservice-over-ssl-in-sharepoint-ssl?blog=1 for helping guide the way.